WiseCashier-SDK for Windows
1. Introduction
1.1 Overview
This document is intended to help developers integrate WiseCashier's payment API on Windows hosts, enabling merchant applications to interact with payment terminals or POS devices. The two devices communicate via WLAN/LAN, ensuring efficient and stable connectivity.
Before reading this document, please first refer to Cross-Terminal Application Integration - WLAN/LAN Mode.
We provide a specially designed Windows SDK for the ECR payment solution, which includes the following files:
File Name | Description |
---|---|
wisesdk_ecr_win.h | This header file contains API function definitions. |
error_code_ecr.h | This header file contains error code definitions. |
WiseSdk_EcrHost_Payment_X86.lib | Import library required by Visual Studio for the x86 platform. |
WiseSdk_EcrHost_Payment_X86.dll | Dynamic library required by the ECR program for the x86 platform. |
WiseSdk_EcrHost_Payment_X64.lib | Import library required by Visual Studio for the x64 platform. |
WiseSdk_EcrHost_Payment_X64.dll | Dynamic library required by the ECR program for the x64 platform. |
1.2 Software Architecture
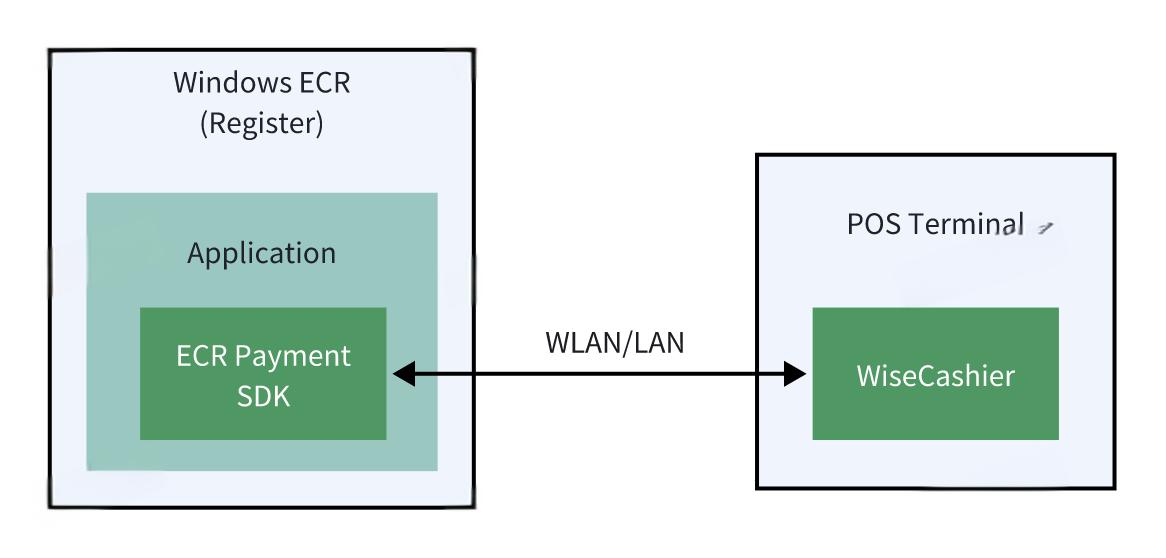
Using WLAN/LAN connectivity, the ECR host and payment terminal must be on the same local network, ensuring normal network communication between them.
2 Development Environment Setup
This section describes the development environment setup process using C# as an example.
2.1 Download and Install Visual Studio
You can download Visual Studio 2017 or later from Microsoft's official website. It is recommended to set Visual Studio's default encoding to "UTF-8 with signature," as shown below:
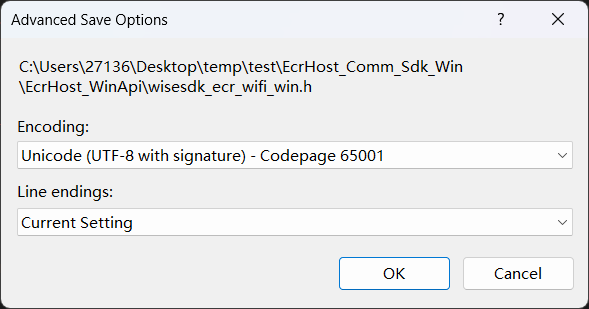
2.2 Create a New Project
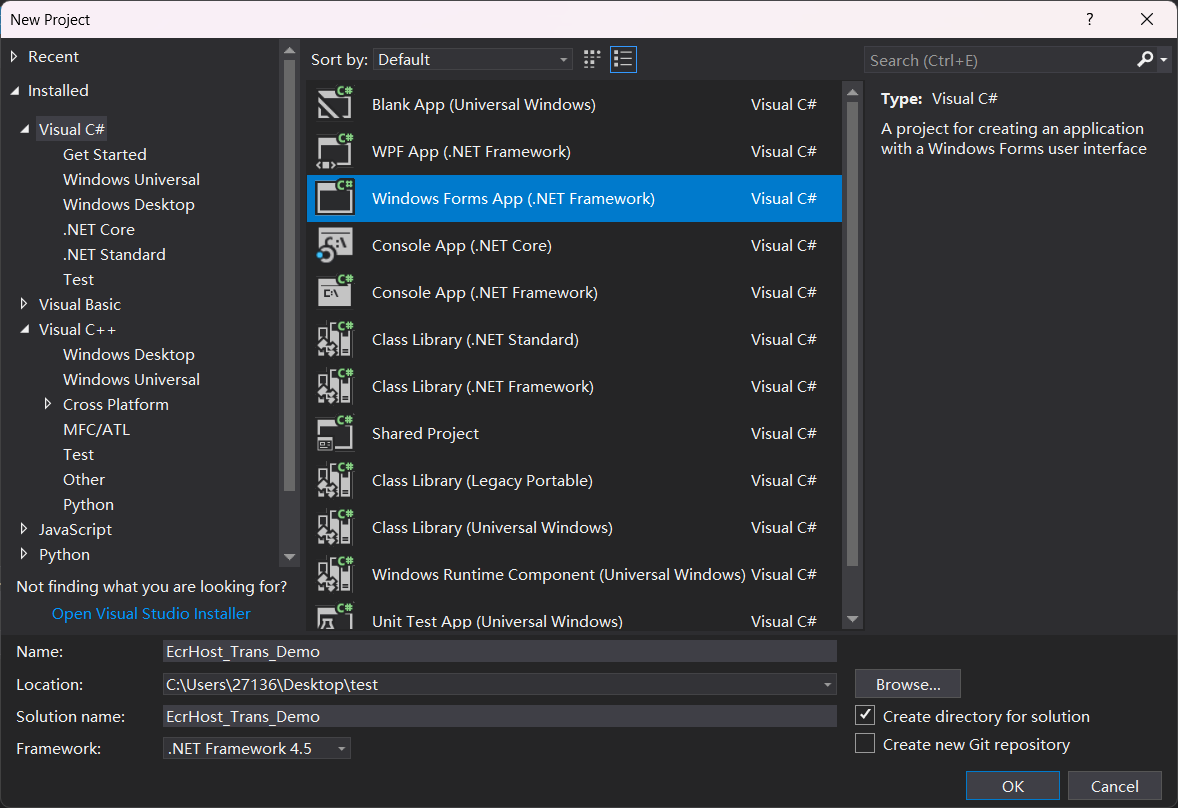
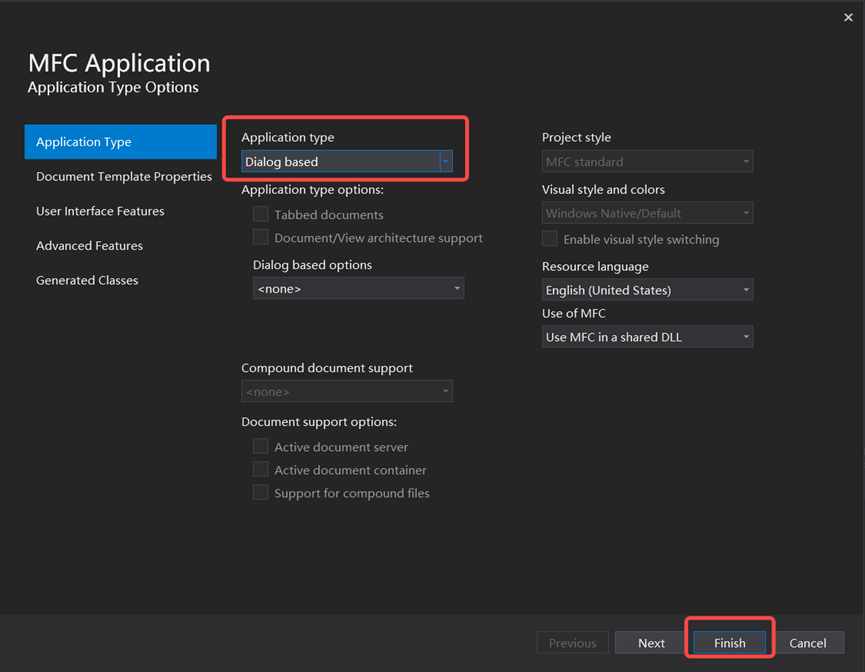
- Copy the DLL files to your execution directory, as shown in the screenshot below:
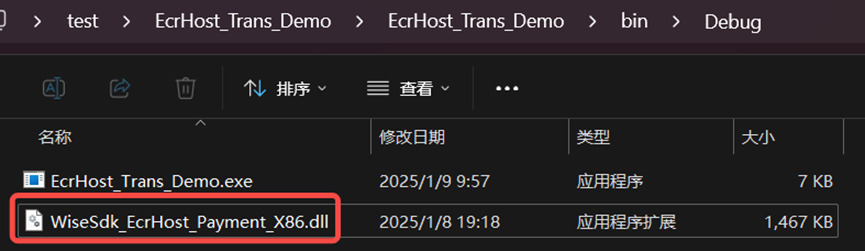
- Import the specified functions into your program.

- Call the functions from the dynamic library to build and run the project.
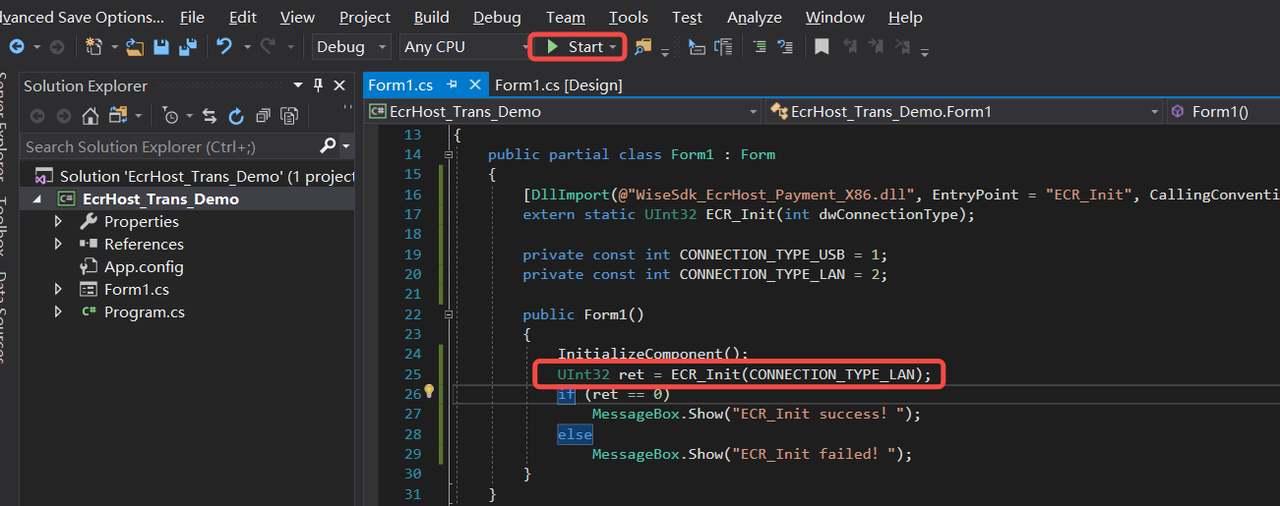
2.3 Import the Demo Project
Open the EcrHost_TransSdk.sln
file using Visual Studio.
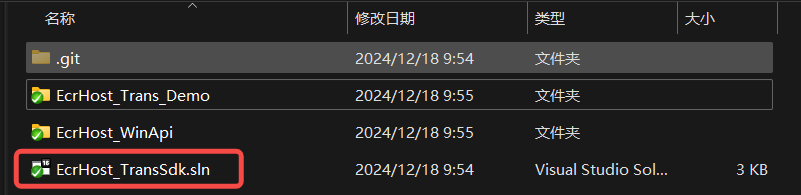
2.4 Run the Project
Click the "Local Windows Debugger" button to run the project.
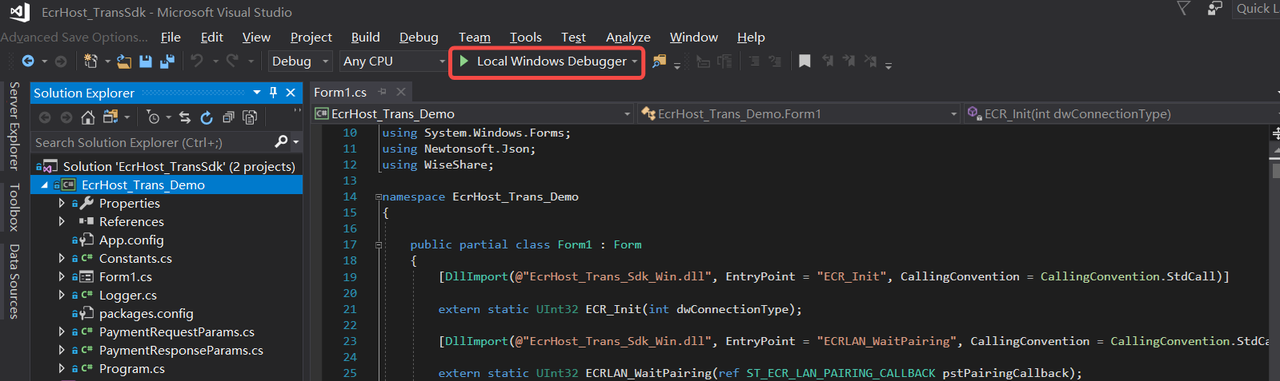
2.5 Connect to the POS Terminal (via Wi-Fi)
- Select "Wi-Fi" mode, click the "Start" button, and wait for pairing. The log area will display the scanned device IP address.
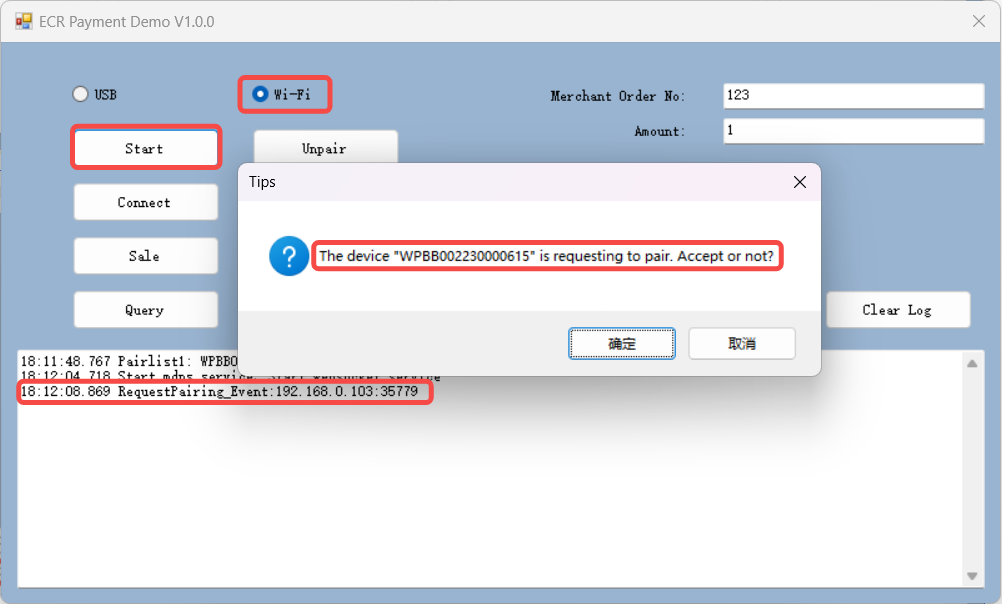
- Once paired, click the "Connect" button.
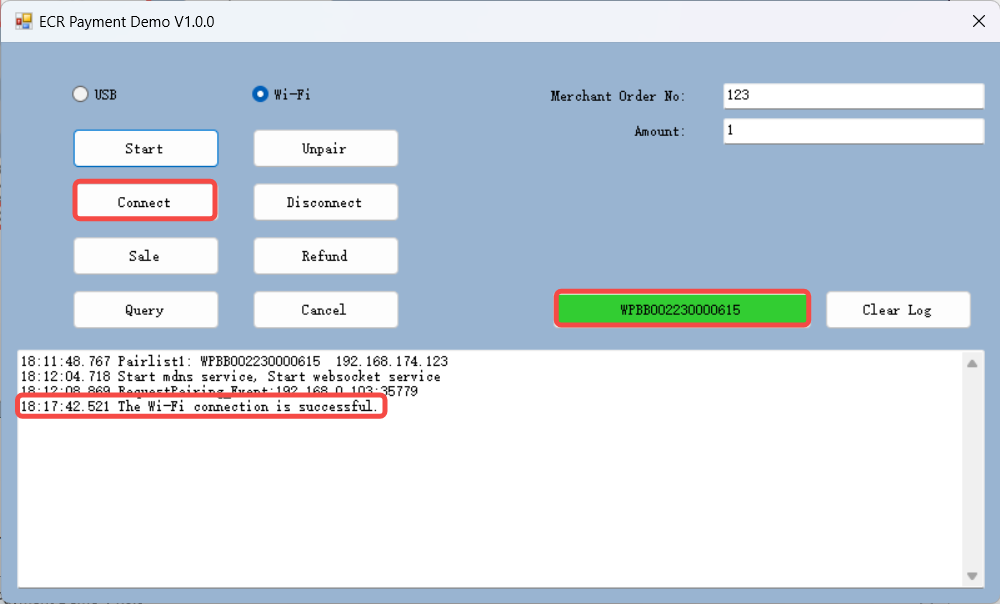
- After a successful connection, enter the "Merchant Order Number" and "Amount," then click the "Sale" button.
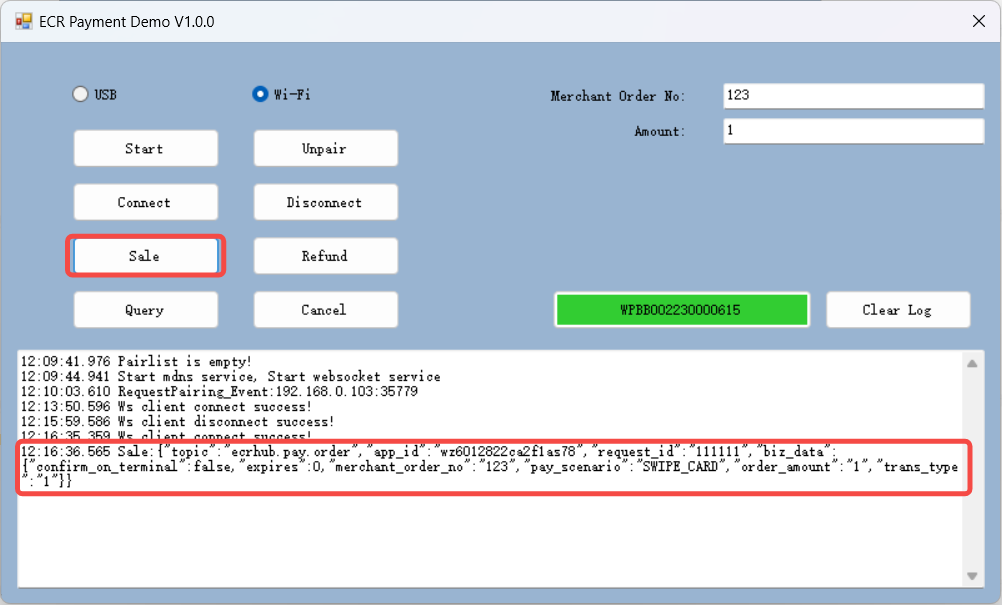
3. Data Structures
3.1 Terminal Information
typedef struct
{
char szTerminalMacAddr[18]; // The MAC address of the terminal, ending with ‘\0’. for example, "00:1A:2B:3C:4D:5E".
char szTerminalName[65]; // The terminal name, ending with ‘\0’.
BYTE bTerminalNameLen; // The length of the terminal name which includes the ‘\0’ terminator.
char szAliasName[65]; // The terminal alias name, ending with ‘\0’.
BYTE bAliasNameLen; // The length of the terminal alias name which includes the ‘\0’ terminator.
char szTerminalIp[40]; // The IP address of the terminal, ending with ‘\0’. for example, "192.168.1.2".
BYTE bTerminalIpLen; // The length of IP address which includes the ‘\0’ terminator.
DWORD dwPort; // The IP port
char szTerminalSn[33]; // The terminal serial number, ending with ‘\0’
BYTE bTerminalSnLen; // The length of the terminal serial number which includes the ‘\0’ terminator.
}ST_TERMINAL_INFO;
4. Process Description
Please note that this flowchart is intended to present a general process, covering key points such as device pairing, network connection, and transaction processing. In actual operation, some API calls and functions do not need to be executed every time. Additionally, some APIs and functions are not shown in this flowchart. You can adjust the process according to your specific requirements.
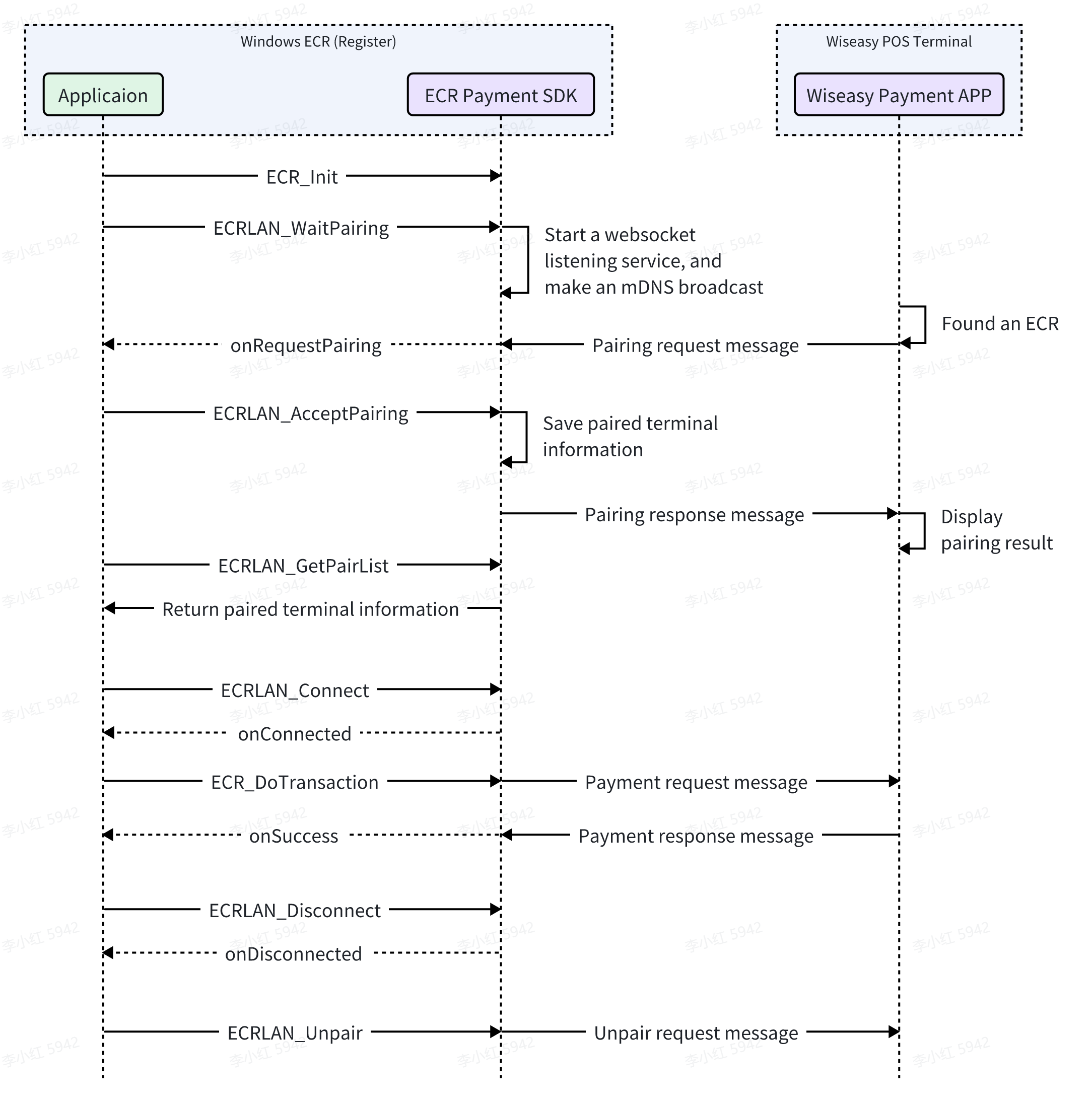
5. APIs
5.1 Sequence
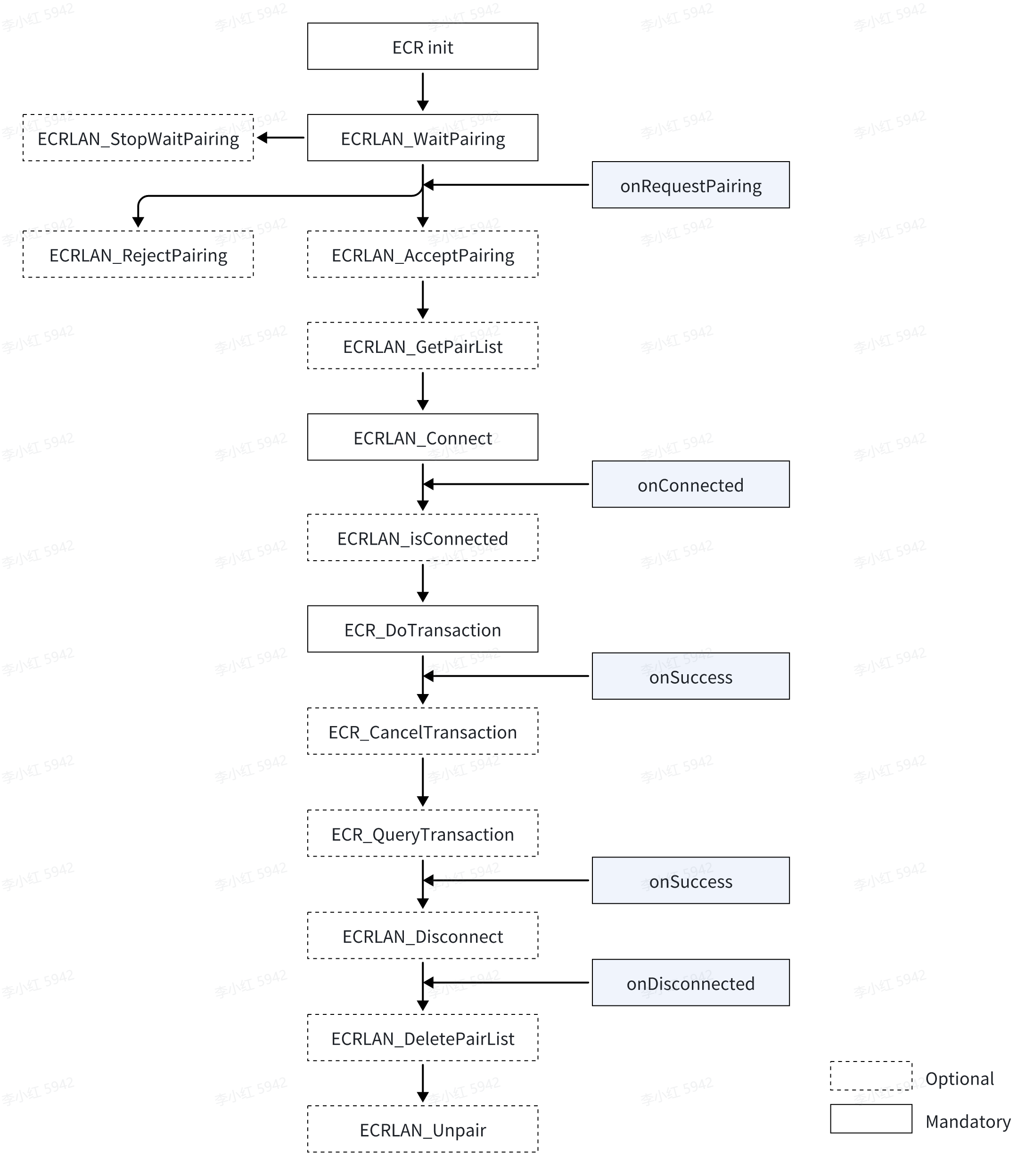
5.2 ECR Initialization
This function is used to initialize the SDK. It performs the necessary internal initialization, and the application must call this function before calling any other functions. However, it only needs to be called once.
DWORD ECR_Init(IN DWORD dwConnectionType);
Parameters | |
---|---|
dwConnectionType | This parameter specifies the communication interface between the ECR Register and the terminal, which can be CONNECTION_TYPE_USB or CONNECTION_TYPE_LAN . |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
UInt32 ret = ECR_Init(CONNECTION_TYPE_LAN);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("ECR Init successful!");
}
5.3 Network Pairing and Connection
5.3.1 Waiting for Pairing
This function is used to establish network pairing between the ECR and the POS terminal. Within this function, an mDNS broadcast is performed, and a WebSocket listening service is started to receive pairing requests from the terminal.
DWORD ECRLAN_WaitPairing(IN ST_ECR_LAN_PAIRING_CALLBACK *pstPairingCallback);
Parameters | |
---|---|
pstPairingCallback | A structure pointer containing multiple callback functions for network pairing. Please refer to the section Network pairing callback. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
ST_ECR_LAN_PAIRING_CALLBACK _lanPairCallback = new ST_ECR_LAN_PAIRING_CALLBACK();
_lanPairCallback.RequestPair = new onRequestPairing(RequestPairing_Event);
_lanPairCallback.RequestUnpair = new onRequestUnpairing(RequestUnpairing_Event);
UInt32 ret = ECRLAN_WaitPairing(ref _lanPairCallback);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("ECRLAN Wait Pairing!");
}
else if (ret == ERR_ECR.ERR_ECR_WAIT_PAIRING)
{
MessageBox.Show("ECRLAN Wait Pair is already running!");
}
5.3.2 Stop Waiting for Pairing
This function is used to stop the initiated pairing process.
DWORD ECRLAN_StopWaitPairing(void);
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
UInt32 ret = ECRLAN_StopWaitPairing();
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("ECRLAN Stop Wait Pairing successful!");
}
5.3.3 Accept Pairing
This function is used to accept the pairing request sent by the terminal. After accepting the terminal's pairing request, the function will internally save the paired terminal's information permanently. Unless the application calls the ECRLAN_DeletePairList
function to delete the pairing information, the pairing data will persist.
DWORD ECRLAN_AcceptPairing(IN ST_TERMINAL_INFO *pstTerminalInfo);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that will be accepted for pairing.Regarding the terminal information structure, please refer to the section Terminal information. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
List<ST_TERMINAL_INFO> _lstStTerminalInfo = new List<ST_TERMINAL_INFO>();
_lstStTerminalInfo = GetPairArray();
if (_lstStTerminalInfo.Count <= 0)
{
MessageBox.Show("Pairlist is empty!");
return;
}
_curTerminalInfo = _lstStTerminalInfo[0];
UInt32 ret = ECRLAN_AcceptPairing(ref _curTerminalInfo);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("ECRLAN Accept Pairing successful!");
}
5.3.4 Reject Pairing
This function is used to reject the pairing request sent by the terminal.
DWORD ECRLAN_RejectPairing(IN ST_TERMINAL_INFO *pstTerminalInfo);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that will be rejected for pairing. Regarding the terminal information structure, please refer to the section Terminal information. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
List<ST_TERMINAL_INFO> _lstStTerminalInfo = new List<ST_TERMINAL_INFO>();
_lstStTerminalInfo = GetPairArray();
if (_lstStTerminalInfo.Count <= 0)
{
MessageBox.Show("Pairlist is empty!");
return;
}
_curTerminalInfo = _lstStTerminalInfo[0];
UInt32 ret = ECRLAN_RejectPairing(ref _curTerminalInfo);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("ECRLAN Reject Pairing successful!");
}
5.3.5 Cancel Pairing
This function notifies the terminal and terminates the pairing relationship with it. However, it does not disconnect the current network connection. Additionally, this function will automatically remove the terminal from the internal pairing list
void ECRLAN_Unpair(IN ST_TERMINAL_INFO *pstTerminalInfo);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that will be unpaired. Regarding the terminal information structure, please refer to the section Terminal information. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
List<ST_TERMINAL_INFO> _lstStTerminalInfo = new List<ST_TERMINAL_INFO>();
_lstStTerminalInfo = GetPairArray();
if (_lstStTerminalInfo.Count <= 0)
{
MessageBox.Show("Pairlist is empty!");
return;
}
_curTerminalInfo = _lstStTerminalInfo[0];
ECRLAN_Unpair(ref _curTerminalInfo);
5.3.6 Get Pairing List
This function is used to retrieve a list of all paired terminal information maintained within the SDK.
DWORD ECRLAN_GetPairList(OUT ST_TERMINAL_INFO *pstTerminalInfo, IN OUT DWORD *pdwNum);
Parameters | |
---|---|
pstTerminalInfo | A pointer to an array of structures used to hold information about terminals that have ever been successfully paired. When this parameter is NULL, this function will return “ERROR_SUCCESS” and the pdwNum parameter will return the actual number of paired terminals. Regarding the terminal information structure, please refer to the section Terminal information. |
pdwNum | As an input parameter, it indicates the maximum expected number of paired terminals to be obtained. If the size of pstTerminalInfo is insufficient, ERR_ECR_BUFFER_NOT_ENOUGH will be returned. As an output parameter, it indicates the number of terminals actually returned that were ever paired successfully. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
ST_TERMINAL_INFO[] curTerminalInfo = new ST_TERMINAL_INFO[20];
int curNum = 20;
IntPtr ptrTerminals = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(ST_TERMINAL_INFO)) * 20);
UInt32 ret = ECRLAN_GetPairList(ptrTerminals, ref curNum);
for (int i = 0; i < curNum; i++)
{
IntPtr ptr = (IntPtr)((UInt32)ptrTerminals + i * Marshal.SizeOf(typeof(ST_TERMINAL_INFO)));
curTerminalInfo[i] = (ST_TERMINAL_INFO)Marshal.PtrToStructure(ptr, typeof(ST_TERMINAL_INFO));
}
Marshal.FreeHGlobal(ptrTerminals);
List<ST_TERMINAL_INFO> lstStTerminalInfo = new List<ST_TERMINAL_INFO>();
for (int i = 0; i < curNum; i++)
{
lstStTerminalInfo.Add(curTerminalInfo[i]);
}
5.3.7 Delete Pairing List
This function is used to remove all terminals or a specified terminal from the pairing information list. It does not require notifying the terminal.
DWORD ECRLAN_DeletePairList(IN ST_TERMINAL_INFO *pstTerminalInfo, IN DWORD dwFlag);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that will be deleted. To delete all paired terminals, set to NULL. Regarding the terminal information structure, please refer to the section Terminal information. |
dwFlag | A flag. If the value is 0, the specified terminal is deleted; if the value is 1, all paired terminals are deleted. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
_lstStTerminalInfo = GetPairArray();
if (_lstStTerminalInfo.Count <= 0)
{
MessageBox.Show("Pairlist is empty!");
return;
}
UInt32 ret = ECRLAN_DeletePairList(_lstStTerminalInfo[0], 0);
if (_ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("ECRLAN Delete PairList successful!");
return;
}
5.3.8 Network Connection
This function is used to establish a network connection with the terminal.
DWORD ECRLAN_Connect(IN ST_TERMINAL_INFO *pstTerminalInfo, IN DWORD dwWaitingSeconds, IN ST_ECR_CONNECTION_CALLBACK *pstConnectionCallback);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that will be connected. Regarding the terminal information structure, please refer to the section Terminal information. |
dwWaitingSeconds | Timeout time in seconds |
pstConnectionCallback | A structure pointer containing multiple callback functions for connection. Please refer to the section Connection callback. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
_lstStTerminalInfo = GetPairArray();
if (_lstStTerminalInfo.Count <= 0)
{
MessageBox.Show("Pairlist is empty!");
return ERR_ECR.ERR_ECR_SUCCESS;
}
_ecrConnectCallback.WsConnected = new onWsConnected(WsConnected_Event);
_ecrConnectCallback.WsDisconnected = new onWsDisconnected(WsDisconnected_Event);
_ecrConnectCallback.WsError = new onWsError(WsError_Event);
_curTerminalInfo = _lstStTerminalInfo[0];
UInt32 ret = ECRLAN_Connect(ref _curTerminalInfo, 60, ref _ecrConnectCallback);
return ret;
5.3.9 Network Disconnect
This function is used to disconnect the network connection with the current terminal.
DWORD ECRLAN_Disconnect(void);
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
UInt32 ret = ERR_ECR.ERR_ECR_SUCCESS;
if (_connectionType == CONNECTION_TYPE_LAN)
ret = ECRLAN_Disconnect();
if (ret != ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show($"ECRLAN_Disconnect Errorcode:{ret.ToString("X2")}");
}
5.3.10 Get Network Connection Status
This function is used to check the network connection status with the terminal.
BOOL ECRLAN_isConnected(void);
Return | |
---|---|
Network connection status | TRUE: the network is connected; FALSE: the network is in a disconnected state. |
Sample code:
if (TRUE == ECRLAN_isConnected())
return ERR_ECR.ERR_ECR_SUCCESS;
5.4 Network Pairing Callback
5.4.1 Definition of Callback Structure
typedef struct
{
//This callback function is called when Terminal requests a pairing
void (*onRequestPairing)(IN ST_TERMINAL_INFO *pstTerminalInfo);
//This callback function is called when Terminal requests to be unpaired
void (*onRequestUnpairing)(IN ST_TERMINAL_INFO *pstTerminalInfo);
}ST_ECR_LAN_PAIRING_CALLBACK;
5.4.2 onRequestPairing
This callback function is called when the terminal requests pairing.
void (*onRequestPairing)(IN ST_TERMINAL_INFO *pstTerminalInfo);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that requests a pairing. Regarding the terminal information structure, please refer to the section Terminal information. |
5.4.3 onRequestUnpairing
This callback function is called when the terminal requests unpairing.
void (*onRequestUnpairing)(IN ST_TERMINAL_INFO *pstTerminalInfo);
Parameters | |
---|---|
pstTerminalInfo | The terminal information of the specified terminal that requests unpairing. Regarding the terminal information structure, please refer to the section Terminal information. |
5.5 Connection Callback
5.5.1 Definition of Callback Structure
typedef struct
{
//This callback function is called when a network or USB connection is established
void (*onConnected)(void);
//This callback function is called when the network or USB connection is disconnected
void (*onDisconnected)(void);
//This callback function is called when an error occurs during connection establishment
void (*onError)(IN DWORD dwErrCode, IN char *pszErrMsg);
}ST_ECR_CONNECTION_CALLBACK;
5.5.2 onConnected
This callback function is called when the network connection is established.
void (*onConnected)(void);
5.5.3 onDisconnected
This callback function is called when the network connection is disconnected.
void (*onDisconnected)(void);
5.5.4 onError
This callback function is called when an error occurs during the process of establishing the connection.
void (*onError)(IN DWORD dwErrCode, IN char *pszErrMsg);
Parameters | |
---|---|
dwErrCode | The error code. |
pszErrMsg | The detailed error message. |
5.6 Transaction
5.6.1 Start Transaction
This function is used to initiate a transaction process on the terminal, including purchases, cashback, voids, pre-authorizations, and more.
DWORD ECR_DoTransaction(IN char *pszRequestMessage, IN DWORD dwWaitingSeconds, IN ST_ECR_TRANS_CALLBACK *pstTransCallback);
Parameters | |
---|---|
pszRequestMessage | Request message data about the transaction sent by the ECR Register to the terminal. The message is in Json format. Please refer to the section Transaction message-Submit Transaction. |
dwWaitingSeconds | The timeout time for the transaction in seconds. If terminal does not return at the set time, WAIT_TIMEOUT is returned. |
pstTransCallback | A structure pointer containing multiple callback functions for transaction. Please refer to the section Transaction callback. In the correct case, onSuccess is called, and the pszResponse parameter returns the terminal's response message (in Json format). In case of error, onError is called. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
ST_ECR_TRANS_CALLBACK _transCallback = new ST_ECR_TRANS_CALLBACK();
_transCallback.TransSuccess = new onTransSuccess(TransSuccess_Event);
_transCallback.TransError = new onTransError(TransError_Event);
PaymentRequestParams reqParams = new PaymentRequestParams();
reqParams.app_id = "wz6012822ca2f1as78";
reqParams.topic = Constants.PAYMENT_TOPIC;
reqParams.biz_data = new PaymentRequestParams.BizData();
reqParams.biz_data.merchant_order_no = tbOrderNumber.Text;// "123" + DateTime.Now.ToString("yyyyMMddHHmmss");
reqParams.biz_data.order_amount = tbAmount.Text;//"11";
reqParams.biz_data.pay_scenario = "SWIPE_CARD";
reqParams.biz_data.trans_type = Constants.TRANS_TYPE_SALE;
string json = JsonConvert.SerializeObject(reqParams, new JsonSerializerSettings { NullValueHandling = NullValueHandling.Ignore });
UInt32 ret = ECR_DoTransaction(json, 60, ref _transCallback);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show($"Sale:{json}");
}
else
{
MessageBox.Show($"Sale ErrorCode:{ret.ToString("X2")}");
}
5.6.2 Cancel Transaction
This function is used to cancel the terminal's execution of a transaction. Please note that not all transactions can be canceled; only those that are in a pending user interaction state can be canceled.
DWORD ECR_CancelTransaction(IN char *pszRequestMessage, IN DWORD dwWaitingSeconds);
Parameters | |
---|---|
pszRequestMessage | Request message data about the transaction sent by the ECR Register to the terminal. The message is in Json format. Please refer to the section Transaction message-Close Transaction. |
dwWaitingSeconds | The timeout time for the transaction in seconds. If terminal does not return at the set time, WAIT_TIMEOUT is returned. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
PaymentRequestParams reqParams = new PaymentRequestParams();
reqParams.app_id = "wz6012822ca2f1as78";
reqParams.topic = Constants.CLOSE_TOPIC;
reqParams.biz_data = new PaymentRequestParams.BizData();
reqParams.biz_data.merchant_order_no = tbOrderNumber.Text; //"123" + DateTime.Now.ToString("yyyyMMddHHmmss");
JsonSerializerSettings jsetting = new JsonSerializerSettings();
jsetting.DefaultValueHandling = DefaultValueHandling.Ignore;
jsetting.NullValueHandling = NullValueHandling.Ignore;
string json = JsonConvert.SerializeObject(reqParams, jsetting);
UInt32 ret = ECR_CancelTransaction(json, 60);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show("Cancel:{json}");
}
else
{
MessageBox.Show($"Cancel ErrorCode:{ret.ToString("X2")}");
}
5.6.3 Query Transaction
This function is used to query the transaction records on the terminal.
DWORD ECR_QueryTransaction(IN char *pszRequestMessage, IN DWORD dwWaitingSeconds, IN ST_ECR_TRANS_CALLBACK *pstTransCallback);
Parameters | |
---|---|
pszRequestMessage | Request message data about the transaction sent by the ECR Register to the terminal. The message is in Json format. Please refer to the section Transaction message-Query Transaction. |
dwWaitingSeconds | The timeout time for the transaction in seconds. If terminal does not return at the set time, WAIT_TIMEOUT is returned. |
pstTransCallback | A structure pointer containing multiple callback functions for transaction. Please refer to the section Transaction callback. In the correct case, onSuccess is called, and the pszResponse parameter returns the terminal's response message (in Json format). In case of error, onError is called. |
Return | |
---|---|
0 | Success |
Other values | Refer to Error Code table |
Sample code:
ST_ECR_TRANS_CALLBACK _transCallback = new ST_ECR_TRANS_CALLBACK();
_transCallback.TransSuccess = new onTransSuccess(TransSuccess_Event);
_transCallback.TransError = new onTransError(TransError_Event);
PaymentRequestParams reqParams = new PaymentRequestParams();
reqParams.app_id = "wz6012822ca2f1as78";
reqParams.topic = Constants.QUERY_TOPIC;
reqParams.biz_data = new PaymentRequestParams.BizData();
reqParams.biz_data.merchant_order_no = tbOrderNumber.Text;
JsonSerializerSettings jsetting = new JsonSerializerSettings();
jsetting.DefaultValueHandling = DefaultValueHandling.Ignore;
jsetting.NullValueHandling = NullValueHandling.Ignore;
string json = JsonConvert.SerializeObject(reqParams, jsetting);
UInt32 ret = ECR_QueryTransaction(json, 60, ref _transCallback);
if (ret == ERR_ECR.ERR_ECR_SUCCESS)
{
MessageBox.Show($"Query:{json}");
}
else
{
MessageBox.Show($"Query ErrorCode:{ret.ToString("X2")}");
}
5.7 Transaction Callback
5.7.1 Definition of Callback Structure
typedef struct
{
//This function is called when a transaction is executed correctly.
void (__stdcall *onSuccess)(IN char *pszResponse);
//This function is called when there is an error in the execution of a transaction.
void (__stdcall *onError)(IN DWORD dwErrCode, IN char *pszErrMsg);
}ST_ECR_TRANS_CALLBACK;
5.7.2 onSuccess
This function is called when the transaction is successful.
void (*onSuccess)(IN char *pszResponse);
Parameters | |
---|---|
pszResponse | Request message data about the transaction sent by the ECR Register to the terminal. The message is in Json format. Please refer to the section Transaction message. |
5.7.3 onError
This function is called when the transaction fails.
void (*onError)(IN DWORD dwErrCode, IN char *pszErrMsg);
Parameters | |
---|---|
dwErrCode | The error code. |
pszErrMsg | The detailed error message. |
6. Appendix
6.1 Error Code Table
Key | Value | 说明 |
---|---|---|
ERR_SUCCESS | 0 | The operation succeeded |
ERR_ECR_INVALID_PARAMETER | 0xE0030001 | Invalid parameter |
ERR_ECR_LENGTH_OUT_RANGE | 0xE0030002 | The length is out of range |
ERR_ECR_DATA_CHECK | 0xE0030005 | Bad data checksum |
ERR_ECR_BAD_DATA | 0xE0030006 | Error in the data |
ERR_ECR_BUFFER_NOT_ENOUGH | 0xE0030007 | Insufficient buffer size |
ERR_ECR_TIMEOUT | 0xE0030008 | Communication timeout |
ERR_ECR_READ_DATA | 0xE003000A | Failed to read data |
ERR_ECR_WRITE_DATA | 0xE003000B | Failed to write data |
ERR_ECR_USB_CABLE_NOT_CONNECTED | 0xE003000F | No terminal connected to ECR via USB cable |
ERR_ECR_USB_NOT_CONNECTED | 0xE0030010 | No USB connection has been established |
ERR_ECR_WIFI_NOT_CONNECTED | 0xE0030011 | No Wi-Fi connection has been established |
ERR_ECR_WIFI_SEND_FAILED | 0xE0030012 | Failed to send data via Wi-Fi |
ERR_ECR_DEV_UNPAIRED | 0xE0030013 | Device unpaired |
ERR_ECR_JSON_ERROR | 0xE0030014 | Json data incorrect |
ERR_ECR_WAIT_PAIRING | 0xE0030015 | It is already in the state of waiting for the pairing request from the terminal |