WiseCashier-SDK for JAVA
This feature is currently under development and is expected to be available in an upcoming release. Thank you for your interest and patience!
1. Getting Started
1.1 Prerequisite
- JDK version 1.8 and above development environment.
- Desktop computer or device running Windows, MacOS or Linux operating systems.
1.2 Installation & Configuration
-
Application installation and settings, please refer to USB mode integration and WLAN/LAN mode integration.
-
Download the jar package and add it to your project, please refer to the GitHub source code.
Source code & Library | WLAN Mode | USB Mode |
---|---|---|
https://github.com/paycloud-open/ecrhub-client-sdk-java | Windows/Linux/MacOS | Windows |
https://github.com/paycloud-open/ecrhub-client-demo-java |
- Maven dependencies
The SDK depends on some open-source third-party jars. If these jars are not integrated into your project, you will need to manually add dependencies to your project.
<!-- Mandatory -->
<!-- jSerialComm -->
<dependency>
<groupId>com.fazecast</groupId>
<artifactId>jSerialComm</artifactId>
<version>[2.0.0,3.0.0)</version>
</dependency>
<!-- WebSocket -->
<dependency>
<groupId>org.java-websocket</groupId>
<artifactId>Java-WebSocket</artifactId>
<version>1.5.4</version>
</dependency>
<!-- fastjson2 -->
<dependency>
<groupId>com.alibaba.fastjson2</groupId>
<artifactId>fastjson2</artifactId>
<version>2.0.26</version>
</dependency>
<!-- hutool -->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.21</version>
</dependency>
<!-- slf4j-api -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>2.0.9</version>
</dependency>
<!-- jmdns -->
<dependency>
<groupId>org.jmdns</groupId>
<artifactId>jmdns</artifactId>
<version>3.5.8</version>
</dependency>
<!-- non-mandatory -->
<!-- logback -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.3.11</version>
</dependency>
<!-- junit -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
2. Function List
2.1 Connect
Select a paired terminal to initiate a network connection, and once the connection is established, transaction instructions can be sent.
2.1.1 Call process
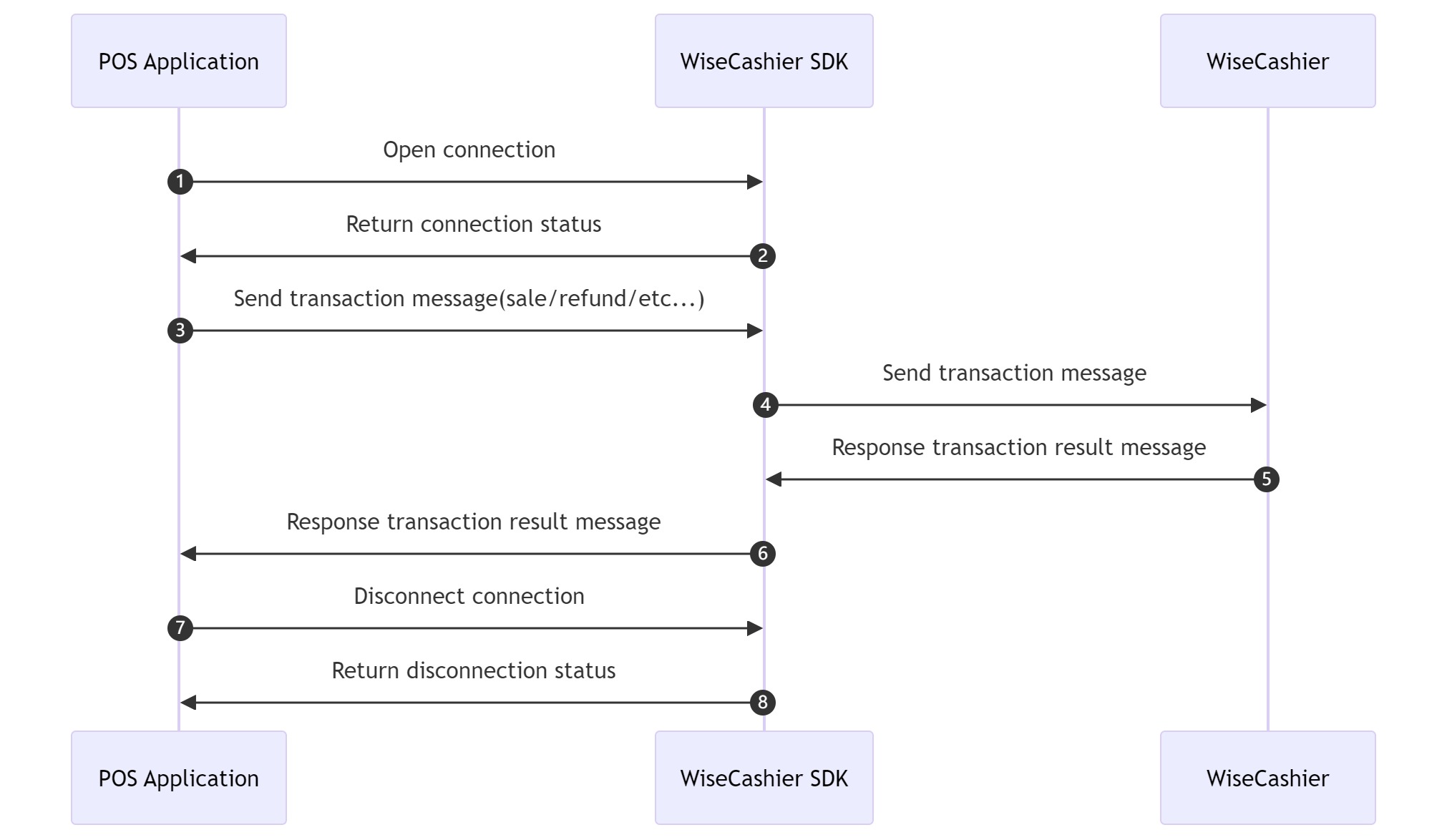
2.1.2 Create client instance
USB connection mode
When a POS application connects to a POS terminal using a USB cable, use the following method to create a client
import com.wiseasy.ecr.hub.sdk.ECRHubClient;
import com.wiseasy.ecr.hub.sdk.ECRHubConfig;
import com.wiseasy.ecr.hub.sdk.ECRHubClientFactory;
// Create a client instance By Serial port
ECRHubConfig config = new ECRHubConfig();
// Setting Serial Port timeout
config.getSerialPortConfig().setConnTimeout(10 * 1000);
config.getSerialPortConfig().setWriteTimeout(10 * 1000);
config.getSerialPortConfig().setReadTimeout(120 * 1000);
// Method 1: Specify the serial port name. Please replace "xxxxxx" with the real serial port name. For example: COM6
// ECRHubClient client = ECRHubClientFactory.create("sp://xxxxxx", config);
// Method 2: Do not specify the serial port name. The SDK will automatically find available serial port
ECRHubClient client = ECRHubClientFactory.create("sp://", config);
2.1.3 Connection
Establish a connection between the POS application and the POS terminal.
// Connecting to the POS Terminal
client.connect();
2.1.4 Disconnect
Disconnect the POS application from the POS terminal.
// This will try disconnect from POS Terminal
client.disconnect();
2.2 Transactions
2.2.1 Sale
- Request/Response parameters
- Example:
import com.wiseasy.ecr.hub.sdk.model.request.SaleRequest;
import com.wiseasy.ecr.hub.sdk.model.response.SaleResponse;
import com.wiseasy.ecr.hub.sdk.enums.EPayScenario;
// Build sale request
PurchaseRequest request = new PurchaseRequest();
request.setApp_id("Your payment appid"); // Setting your payment application ID
request.setMerchant_order_no("O123456789");
request.setOrder_amount("1");
request.setPay_scenario(EPayScenario.SWIPE_CARD.getVal());
// Setting read timeout,the timeout set here is valid for this request
ECRHubConfig requestConfig = new ECRHubConfig();
requestConfig.getSerialPortConfig().setReadTimeout(2 * 60 * 1000);
request.setConfig(requestConfig);
// Execute sale request
System.out.println("Sale Request:" + request);
PurchaseResponse response = client.execute(request);
System.out.println("Sale Response:" + response);
2.2.2 Refund
- Request/Response parameters
- Example:
import com.wiseasy.ecr.hub.sdk.model.request.RefundRequest;
import com.wiseasy.ecr.hub.sdk.model.response.RefundResponse;
// Build refund request
RefundRequest request = new RefundRequest();
request.setApp_id("Your payment appid"); // Setting your payment application ID
request.setOrig_merchant_order_no("O1695032342508");// The merchant order number of the original order
request.setMerchant_order_no("O123456789");
request.setOrder_amount("1");
// Execute refund request
System.out.println("Refund Request:" + request);
RefundResponse response = client.execute(request);
System.out.println("Refund Response:" + response);
2.2.3 Query
- Request/Response parameters
- Example:
import com.wiseasy.ecr.hub.sdk.model.request.QueryRequest;
import com.wiseasy.ecr.hub.sdk.model.response.QueryResponse;
// Build query request
QueryRequest request = new QueryRequest();
request.setApp_id("Your payment appid"); // Setting your payment application ID
request.setMerchant_order_no("O123456789");
// Execute query request
System.out.println("Query Request:" + request);
QueryResponse response = client.execute(request);
System.out.println("Query Response:" + response);
2.2.4 Close
- Request/Response parameters
- Example:
import com.wiseasy.ecr.hub.sdk.model.request.CloseRequest;
import com.wiseasy.ecr.hub.sdk.model.response.CloseResponse;
// Build close request
CloseRequest request = new CloseRequest();
request.setApp_id("Your payment appid"); // Setting your payment application ID
request.setMerchant_order_no("O123456789");
// Execute close request
System.out.println("Close Request:" + request);
CloseResponse response = client.execute(request);
System.out.println("Close Response:" + response);